Published
- 3 min read
Solving Advent of Code 2023 in Fan
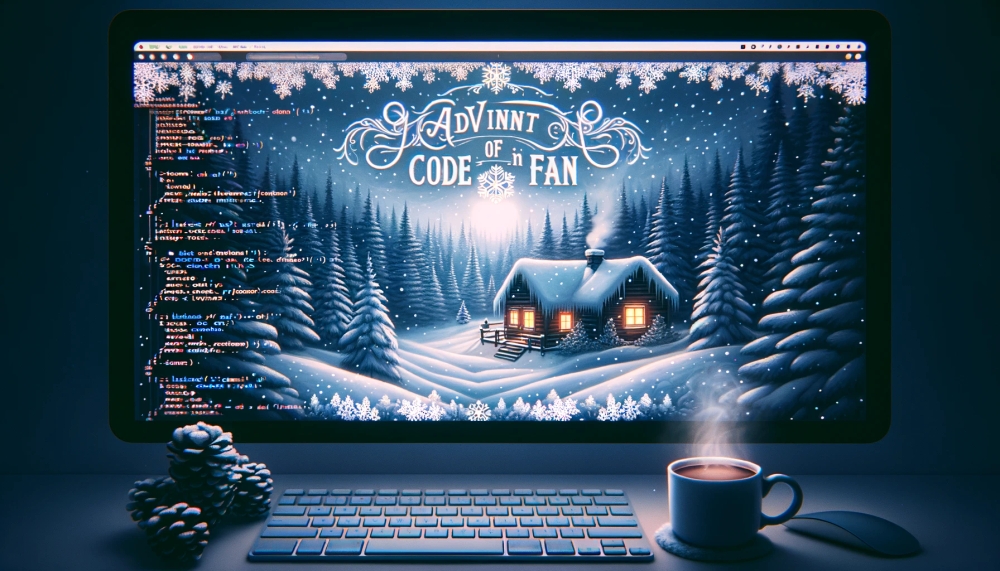
[Advent of Code](https://adventofcode.com) (AOC) is an annual Christmas-themed programming challenge where every day in December until Christmas a new two-part challenge is released. They’re great if you’re looking for a fun challenge, or want to practice a new language. Including, one you wrote yourself.
I’m still on day two because I’m using Advent of Code to test my language and contribute new features to the standard library. However, I did complete day one, and I thought it’s a good example of how a real Fan program works.
import "std/fs" for Fs
import "std/fmt" for Fmt
// import "std/os" for Runtime
var textNum = Fn.new { | input, reverse |
if (!(input is String)) {
Fiber.abort("Paramater must be of type String")
}
// This is where I'd make the input all lowercase if Fan had that in its standard library 😂
if (reverse) {
input = Fmt.reverse(input)
}
// System.print("Input: %(input)")
var index = [
"zero",
"one",
"two",
"three",
"four",
"five",
"six",
"seven",
"eight",
"nine"
]
var keys = {
"zero": 0,
"one": 1,
"two": 2,
"three": 3,
"four": 4,
"five": 5,
"six": 6,
"seven": 7,
"eight": 8,
"nine": 9
}
for (i in index) {
if (input.contains(i)) {
return keys[i]
}
}
return null
}
var input = Fs.read("./1/input.txt")
var output = []
for (line in input.split("\n")) {
var fromStart = Fiber.new {
var buffer = ""
for (i in line) {
if (buffer.count >= 3) {
var strKeys = textNum.call(buffer, false)
if (strKeys is Num) {
// System.print("Found \e[0;32m%(strKeys)\e[0m")
Fiber.yield(strKeys)
}
}
var diget = Num.fromString(i)
if (diget != null) {
Fiber.yield(diget)
}
buffer = buffer + i
}
}
var fromEnd = Fiber.new {
var buffer = ""
var size = line.count
while (size != 0) {
if (buffer.count >= 3) {
var strKeys = textNum.call(buffer, true)
if (strKeys is Num) {
// System.print("Found \e[0;32m%(strKeys)\e[0m")
Fiber.yield(strKeys)
}
}
var letter = line[size - 1]
var diget = Num.fromString(letter)
if (diget != null) {
Fiber.yield(diget)
}
buffer = buffer + letter
size = size - 1
}
}
if (!fromStart.isDone && !fromEnd.isDone) {
var start = fromStart.call()
var end = fromEnd.call()
System.print("\e[0;32m%(start)\e[0m %(line) \e[0;32m%(end)\e[0m")
var target = "%(start)%(end)"
var diget = Num.fromString(target)
if (diget != null) {
output.add(diget)
}
}
}
var final = 0
for (int in output) {
final = final + int
}
System.print("--------------------------------")
System.print(final)
This is a complete program and has been validated for AOC 2023 Day one, both parts one and two. Feel free to test it on your own input.
Other Updates
- Finally figured out Valgrind, so Fan is officially free of memory leaks
- Added std/fmt, which is intended to spice up string manipulation. It’s pretty small right now, but has a fun and fresh Fmt.reverse(_) method.
- Added Color to std/fmt . Currently, it’s a complete reference for ANSI color codes. You can use it in your programs with string interpolation.
System.print("%(Color.green())Hi!%(Color.reset())")
prints:
! I’d love to see what kind of ideas you’ve come up with.